Every project has a development standard
Sometimes the standard is “if it was hard to write, it should be hard to maintain.”
Developing, and following, a corporate Best Practices standard will lead to continuity, maintainability, robustness, and pride.
Coding standards are similar to writing in accordance with APA or MLA. It allows people to focus on content, not formatting.
This information is part of a larger presentation. The original presentation was at the Houston TechFest during the September 2016 event. Please consider adding this to your user group!
Conference Schedule
Wars have been fought … Lives have been lost
Coding Standards Make Software:
- Safe
can be used without causing harm - Secure
can’t be hacked - Reliable
functions as it should, every time - Testable
can be tested at the code level - Maintainable
can be maintained, even as your codebase grows - Portable
works the same in every environment
Coding Standards Make Products:
- Compliant
with industry standards - Consistent
with corporate products and offerings - Secure
from the start - Economic
to developed - Accelerated
time to market
Coding Standards ARE:
- A set of guidelines
- for a specific programming language
- for programming styles, practices, and methods
- for software structural quality
- Applicable to the human maintainers and peer reviewers
- May [should] be formalized and followed by an entire team or company
Coding Standards Address:
- file organization
- indentation
- comments
- declarations
- statements
- white space
- naming conventions
- programming practices
- programming principles
- programming rules of thumb
- architectural best practices, etc.
Coding Standards: Code Stability and Robustness:
- Top 15 Best Practices
- Commenting & Documentation
- Consistent Indentation
- Avoid Obvious Comments
- Code Grouping
- Consistent Naming Scheme
- SOLID / DRY / YAGNI Principles
- Avoid Deep Nesting
- Limit Line Length
- File and Folder Organization
- Consistent Temporary Names
- Capitalize SQL Special Words
- Separation of Code and Data
- Alternate Syntax Inside Templates
- Object Oriented vs. Procedural
- Read Open Source Code
Fundamental Principals:
- SOLID
- Single-responsibility Principle
- Open-closed Principle
- Liskov substitution principle
- Interface segregation principle
- Dependency Inversion principle
- DRY
- Don’t Repeat Yourself
- YAGNI
- Ya Ain’t Gonna Need It
Common Design Patterns:
- Singleton
- Factory
- Iterator
- Abstract
- CQRS
- IOC / DI
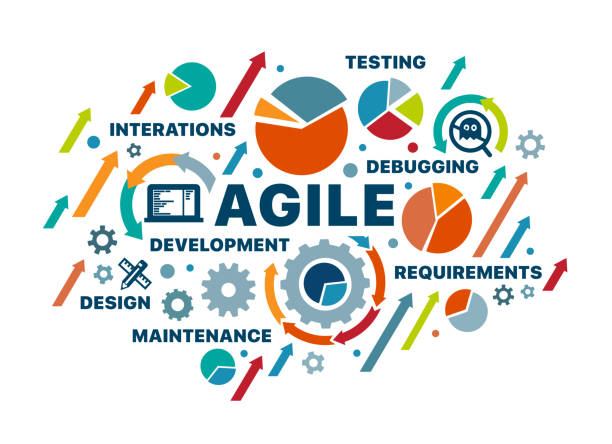
Best Practices Become Agile Software Programming:
- Test-first programming (or perhaps Test-Driven Development),
- Rigorous, regular refactoring,
- Continuous integration,
- Simple design,
- Pair programming,
- Sharing the codebase between all or most programmers,
- A single coding standard to which all programmers adhere,
- A common “war-room” style work area. (no consensus)
Naming Convention:
- Examples:
- Trace a variable from source to grave
- Column in database: Flymm_Flam
- Property in ORM class: Flymm_Flam ( or FlymmFlam ? )
- Represented a local variable: flymmFlam
- Class-Private field: _flymmFlam
- Constant: FLYMM_FLAM ( avoid constants )
- Controls: btnFlymmFlam ( no consensus )
- Method parameter: flymmFlam
Guidelines to Live By:
- DO NOT use single letter variable – spell out the purpose
- Be environment aware – DO NOT use reserved words
- Avoid abbreviations
- Use lastName, not nam
- Unless they are well known like Xml, Html or IO
- DO NOT vary variables by case! (C#)
Maintainability:
- Refactor for code reuse, low cyclomatic complexity values
- If you can’t see your code on one screen in the editor, then it’s probably too long!
- Keep generics in mind
- There are two kinds of programmers:
- Complexifiers are averse to reduction
- Simplifiers thrive on concision
- If your method, class etc. seems huge or overly complicated, then you are most likely doing it wrong!
- Refactor!
- Ask a follow programmer!
- Pair programming!
- Code review!
Tools to add to Visual Studio:
- ReSharper
http://www.jetbrains.com/ - Refactor Pro! For Visual Studio
https://www.devexpress.com/Products/CodeRush/refactor_pro.xml - StyleCop
https://stylecop.codeplex.com/ - CodeIt.Right
http://submain.com/products/codeit.right.aspx - VS Analyze or FXCop
https://msdn.microsoft.com/en-us/library/bb429476(v=vs.80).aspx
Reference Information:
- Design Guidelines for Class Library Developers
http://DGForClassLibrary.notlong.com - .NET Framework General Reference Naming Guidelines
http://namingguide.notlong.com
References:
- 2012, .NET Coding Standards For The Real World , David McCarter
http://www.slideshare.net/dotNetDave/net-coding-standards-for-the-real-world2015, Drupal Coding Standards, Joe Shindelar
http://www.pluralsight.com/courses/drupal-coding-standards2019, Coding Standards For Quality and Compliance, Perforce
https://www.perforce.com/resources/qac/coding-standards2011, Top 15+ Best Practices for Writing Super Readable Code, Burak Guzel
http://code.tutsplus.com/tutorials/top-15-best-practices-for-writing-super-readable-code--net-8118